Improve Signalr and React Performance User Experience
With the last two articles, we now have a working app that shows everyone’s cursor in real time. But before we deploy this to production, let’s do some much needed performance optimizations!
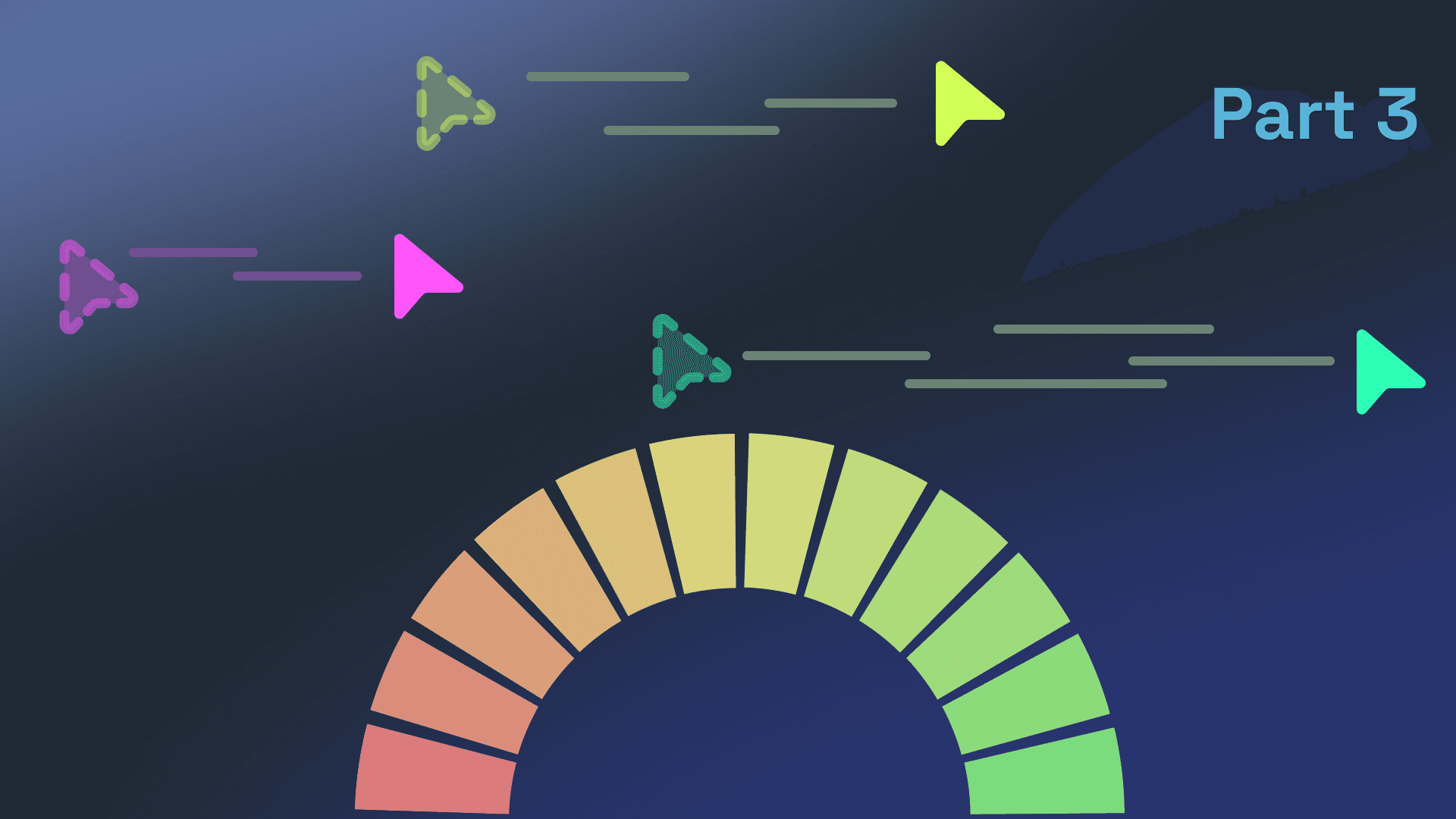
This article is part of my series “Realtime webapps with SignalR and React”:
- Realtime Cursor Tracking with .NET and React using SignalR
- Sync React with SignalR Events
- Improve Signalr and React Performance User Experience (You are here)
Too Many Updates per Second
Modern monitors and graphics cards can handle over 60hz, with some even reaching 240hz. This means the cursor moves between 60 to 240 times per second, causing each client to send updates up to 240 times per second over the SignalR connection.
It’s easy to see this doesn’t scale well. We can definitely sacrifice some of the buttery-smooth cursor movement for scalability and performance. For example, Miro allegedly updates just four times per second.
One way to solve this is by throttling
our mousemove
event listener. Throttling limits how often the event handler runs, ensuring it only executes at set intervals.
Since we might have different throttle requirements in the future, it is best to do this using a higher-order function instead of changing the actual event listener:
1// utils.js2const Throttle = (fn, delay) => {3let ready = true;45// return the throttled function6return (...args) => {7if (!ready) {8return; // block the function if it's not ready9}1011ready = false; // block the function after this call12fn(...args);13setTimeout(() => {14ready = true; // allow the function to be called again after the delay15}, delay);16};17};
A few JavaScript-specific things are happening here:
- The higher-order function takes another function
fn
and a delay timedelay
. - A
ready
variable is set outside the returned function to track when it can run again. - Using an arrow function ensures
this
is captured correctly, so we can useready
inside the throttled function. - The returned function spreads the current arguments and calls
fn(.
.. . args)
By using this higher-order function, we can easily adjust the throttle settings without touching the core logic of the event listener.
Finally, we can wrap our updateCursorPosition
function in the useUpdateCursorLocation
hook with our new Throttle
function like this:
1//normal event handler2const updateCursorPosition = (ev: MouseEvent) => {3// ...function body4};56//throttled event handler7const updateCursorPosition = Throttle(8(ev: MouseEvent) => {9// ...function body10}, 250);
Improving User Experience with Springs
After applying throttling, you’ll notice the user experience has become quite jarring. Instead of smooth movement, the other cursors seem to jump around the screen at noticeable intervals.
While we can’t update the cursor position 60-240 times per second to recreate the exact movements of the other cursors, we can improve the user experience by transitioning smoothly between each updated position.
I got the best results using springs. Springs are an animation technique that don’t rely on a timeline and predefined curves but instead mimic physical properties like stiffness, mass, and damping. The folks over at liveblocks.io dive deeper into different animations and their pros and cons for multiplayer cursor animation in this article.
There are several libraries that provide spring animations. Two of the most popular are react-
and framer-
. Since my projects often need more than just spring animations, I prefer using framer-
.
Adding framer-
is straightforward. We simply replace the div
in our OtherCursor
component with motion.
like this:
1// Feel free to change the settings to suit your animation preference2const spring = {3type: "spring",4damping: 30,5mass: 0.8,6stiffness: 350,7}89export function OtherCursor({ color, name, x, y }: OtherCursorProps) {10return (11<motion.div12style={{ background: color, position: "absolute"}}13initial={{ x, y }}14animate={{ x, y }} // framer-motion now handles the position of each cursor15transition={spring}16>17<div>{name}</div>18</motion.div>19);20}
With this, our mouse movement looks a lot smoother. Personally, I still prefer updating the mouse positions more than 4 times per second since a 250ms delay still looks a bit janky. But depending on your use cases, hardware and performance requirements, this approach works well. If Miro can get away with it, you probably can too.
Wrapping up
Last time, in Sync React with SignalR Events, we connected a React app to a .NET SignalR Hub for realtime cursor tracking. While that solution worked, it wasn’t quite production-ready. Having 60 to 240 cursor updates per second from multiple clients is a bit overkill and not even necessary.
In this article we took control of the updates-per-second by implementing a throttle function. With throttling, we can set a millisecond threshold to limit how often a function can fire.
While this solved performance issues, it hurt the user experience. By introducing spring-based animations, we brought back smooth mouse movement and improved the user experience.
That’s it for now, see you in the next one!
What to read next:
I really hope you enjoyed this article. If you did, you might want to check out some of these articles I've written on similar topics.
- Read Sync React with SignalR Events— 8 min read readSync React with SignalR Events
- Read .NET Aspire & Next.js: The Dev Experience You Were Missing— 7 min read read.NET Aspire & Next.js: The Dev Experience You Were Missing
- Read Build AI-Powered Applications with Microsoft.Extensions.AI— 9 min read readBuild AI-Powered Applications with Microsoft.Extensions.AI